Publish your newsletter to your Remix site with the ConvertKit API
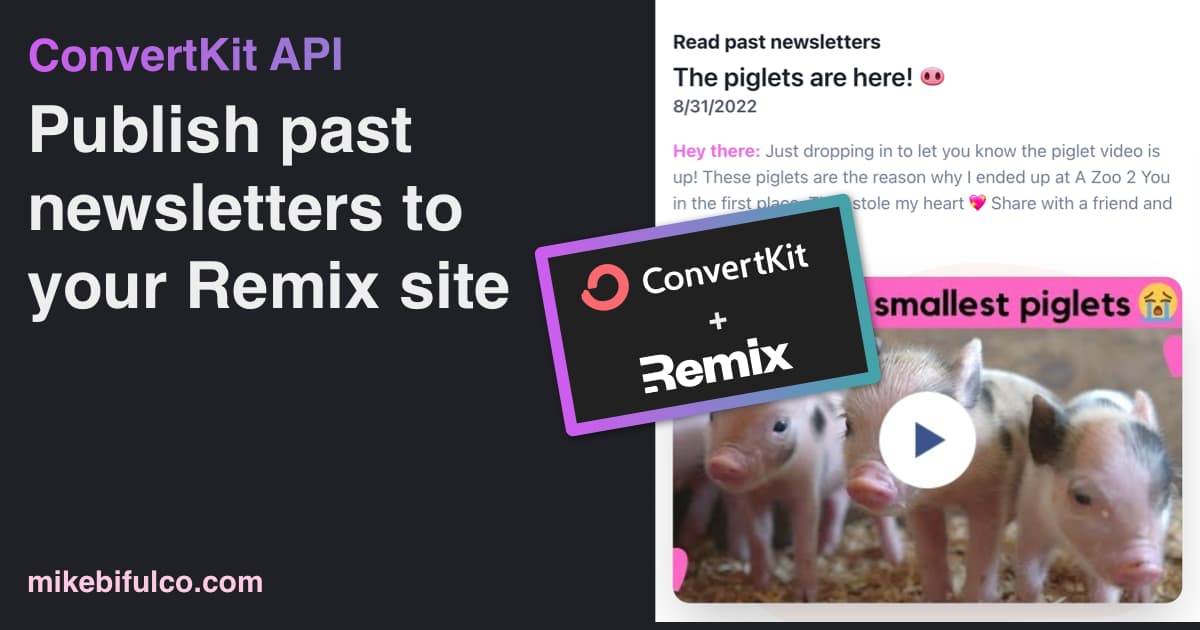
I publish a newsletter called Tiny Improvements
My little newsletter is all about making small, incremental improvements to your work and home life. I've been publishing it for over a year now and I've learned a lot about how to make it better.
It's a great way to share less-formal insights with the people who have given me access to their inbox - a privilege which I don't take lightly. It also helps me keep my writing skills sharp, and it's a great way to get direct feedback on my ideas.
Although the audience for my newsletter has been growing, and recently hit some milestones, I realized that I had quite a bit of great writing that went out once, via email, and was never seen again. I wanted to make it easier for people to find and read my past newsletter content without subscribing, as way to show the value it provides. I also wanted to take advantage of the SEO benefits of having past issues of my newsletter content indexed by search engines.
In this post, we'll go over how I use the ConvertKit API to publish a newsletter page for a site built with Remix.
Improve discoverability by publishing past issues
I have a second, important use case for publishing newsletters online. I also maintain my wife's website - primaryfocus.tv - which she uses to promote her business and YouTube channel (go subscribe!).
Primary Focus has a newsletter, and I wanted to make it easy for her to publish her newsletter to her website as well. In fact, I wanted to give her a way to get her newsletter on her website with zero effort - and I was pleased to find that I could do that with the ConvertKit API.
Ideally, the workflow should look like this: newsletters are drafted and published in ConvertKit, and, once sent to her subscribers, automatically published to the website as well. This way, she can focus on writing and sending her newsletter, without adding an additional workflow to update her site.
Her site is built with Remix, so this post will go over the details of implementation for publishing past ConvertKit newsletters to a remix site, using their API.
Using the ConvertKit API to publish newsletters to a Remix site
Setting up an automated newsletter page is fairly simple, at least in theory:
- Write newsletters and send them, using ConvertKit's broadcast features (for the sake of this post, we'll use the term "newsletter" and "broadcast" somewhat interchangeably).
- Use the ConvertKit API's list-broadcasts endpoint to query for every published broadcast, to populate a newsletter index page
- For each published broadcast, use the ConvertKit API to get the details of that broadcast, and then render the content of that broadcast on a page.
To get newsletters from the ConvertKit API, I set up 2 helper functions. First is getNewsletter(), which takes a broadcast ID and returns the details of that broadcast.
1export const getNewsletter = async (broadcastId) => {2const res = await fetch(3`https://api.convertkit.com/v3/broadcasts/${broadcastId}?api_secret=${CONVERTKIT_API_SECRET}`4);56// rename "broadcast" to "newsletter" for consistency7const { broadcast: newsletter } = await res.json();89return newsletter;10};
The second is getAllNewsletters(), which returns a list of all published broadcasts. In its simplest form, this function looks like this:
1export const getAllNewsletters = async () => {2const response = await fetch(3`https://api.convertkit.com/v3/broadcasts?api_secret=${CONVERTKIT_API_SECRET}`4);5const data = await response.json();67// rename "broadcasts" to "newsletters" for consistency8const { broadcasts: newsletters } = data;910return newsletters;11};
Keeping your ConvertKit API Secret key safe on Remix sites
Remix provides a has a handy filename convention to ensure certain code only runs serverside - any file that ends in .server.ts (for TypeScript) and .server.js (for JavaScript) will only be run on the server. This is perfect for keeping our ConvertKit API secret safe. I created a file called /app/lib/util/convertkit.server.js with the two functions above, to make sure to only ever access the secret key in there.
Rendering the newsletter index page
Now that we have a way to get a list of all published newsletters, we can use that to render a page with a preview of each past issue. I created a new file called /app/routes/newsletter/index.jsx to render the newsletter index page.
note: I stripped out the styling/presentation code from this example to keep it focused on data loading logic.
1import { useLoaderData } from '@remix-run/react';2import { NewsletterCTA, NewsletterListItem } from '~/components';3import { getAllNewsletters } from '~/lib/util/convertKit.server';45export const loader = async ({ params, request }) => {6return {7newsletters: await getAllNewsletters(),8};9};1011const NewsletterListPage = () => {12const { newsletters } = useLoaderData();1314return (15<>16<h1>Read past newsletters</h1>17{newsletters.map((newsletter) => (18<NewsletterListItem newsletter={newsletter} key={newsletter.id} />19))}2021{/* make sure to add a "hey please subscribe to the newsletter" widget to this page! */}22<NewsletterCTA />23</>24);25};2627export default NewsletterListPage;
This is a fairly standard implementation, if you're familiar with Remix. We use the loader pattern to fetch the list of newsletters, and pass them to the render function for the component with the useLoaderData() hook. We then render a list of NewsletterListItem components, which shows a header image and title for each newsletter, along with a link to the page for that specific newsletter.
Rendering a single newsletter page
Now that we have a page listing all published newsletters, we need to create a page template to render a single newsletter. I created a new file called /app/routes/newsletter/$id.jsx to render the newsletter index page (note, once again, that presentation and styling code is removed here for simplicify):
1import { useLoaderData } from '@remix-run/react';2import NewsletterCTA from '~/components';3import config from '~/config';4import {5broadcastTemplateParse,6newsletterHasValidThumbnail,7} from '~/lib/util/convertKit';8import { getNewsletter } from '~/lib/util/convertKit.server';910// render meta tags on page for SEO11export const meta = ({ data }) => {12const { newsletter, canonical } = data;13const publishedAt = new Date(newsletter.published_at);1415const description = `Primary focus newsletter: ${16newsletter.subject17}, published on ${publishedAt.toLocaleDateString()}`;1819return {20description,21title: newsletter.subject22? `${newsletter.subject} - ${config.meta.title}`23: config.meta.title,24'og:image': newsletterHasValidThumbnail(newsletter)25? newsletter.thumbnail_url26: null,27'og:type': 'article',28'og:title': newsletter.subject,29'og:description': description,30'og:url': canonical,31'twitter:card': 'summary_large_image',32};33};3435export const loader = async ({ params, request }) => {36const { id } = params;37const newsletter = await getNewsletter(id);3839return { newsletter };40};4142const NewsletterPage = () => {43const { newsletter } = useLoaderData();4445const publishedAt = new Date(newsletter.published_at);46return (47<>48<h1>{newsletter.subject}</h1>49<p className="publish-date">{publishedAt.toLocaleDateString()}</p>50<div51dangerouslySetInnerHTML={{52__html: broadcastTemplateParse({ template: newsletter.content }),53}}54/>55<NewsletterCTA />56</>57);58};5960export default NewsletterPage;
Once again, we use the loader pattern to query the ConvertKit API for details about this specific newsletter, and pair that with useLoaderData() to provide that information to the page render function. We also use the meta function pattern to render meta tags for SEO purposes - you can read more about that in the Remix docs.
Render the body using dangersoulySetInnerHTML
To render the contents of the newsletter, we are using a scary-looking React API pattern - dangerouslySetInnerHTML. This is a React API that allows you to render HTML that you have received from an API, and is generally considered a security risk. However, in this case, we are using it to render HTML that we have generated ourselves, and we are at least confident that it is somewhat safe to render. Generally speaking, this is a technique that shouldn't be used unless you are confident that the HTML you are rendering is safe.
Helper functions for displaying ConvertKit newsletter content
We're also using 2 helper functions, which are defined in /app/lib/util/convertKit.js (note that these are not in convertkit.server.js, since we need them on the client side):
1import { Liquid } from 'liquidjs';23const engine = new Liquid();45export const broadcastTemplateParse = ({ template, data }) => {6const t = template.replaceAll('"', '"');7const res = engine.parseAndRenderSync(t, data);8return res;9};1011export const newsletterHasValidThumbnail = (newsletter) => {12const { thumbnail_url: thumbnailUrl } = newsletter;13if (!thumbnailUrl) return false;14if (thumbnailUrl.startsWith('https://functions-js.convertkit.com/icons'))15return false;16return true;17};
The newsletterHasValidThumbnail function is a helper function that checks if the newsletter has a valid thumbnail image. ConvertKit provides a default thumbnail image for all newsletters, but you can also upload your own, by adding an image to your newsletter's body. This function checks if the thumbnail is the default image, and if so, returns false so we can skip rendering it.
The broadcastTemplateParse function is a helper function that takes a newsletter template and parses it with the Liquid templating language. This is how ConvertKit allows you to customize the HTML of your newsletter, and use dynamic data like the subscriber's name, or the date the newsletter was sent.
An important caveat: There are lots of little cases where this might not work perfectly, due to all of the amazing things you can do with Liquid in ConvertKit. At the moment, my newsletter uses fairly basic liquid customization - this may not do the trick for you if you're using more advanced features. Please test your implementation thoroughly!
Adding more detail to the index page
At the time of writing this post, ConvertKit's v3 API is in "active development" - and as such, it's likely not feature complete. For example, the current API for list-broadcasts provides a minimal amount of data about each broadcast:
1curl https://api.convertkit.com/v3/broadcasts?api_secret=<your_secret_api_key>
1{2"broadcasts": [3{4"id": 1,5"created_at": "2014-02-13T21:45:16.000Z",6"subject": "Welcome to my Newsletter!"7},8{9"id": 2,10"created_at": "2014-02-20T11:40:11.000Z",11"subject": "Check out my latest blog posts!"12},13{14"id": 3,15"created_at": "2014-02-29T08:21:18.000Z",16"subject": "How to get my free masterclass"17}18]
Since we want to create an index page that shows a thumbnail for each newsletter, we need to query the API for more information about each newsletter. At the moment, the best way to do this is to query the API for each newsletter individually. It's not ideal, since we need to send an API call for each newsletter shown on the index page, but I'm hoping that with a bit of feedback, ConvertKit will add more data to the list-broadcasts API endpoint.
We already have a function to query the API for a single newsletter (remember getNewsletter from earlier?), so we can use that to query the API for each newsletter on the index page.
Filter out un-published newsletters
The list-broadcasts endpoint also doesn't currently return whether or not a given broadcast was published yet - which means that if you draft a future broadcast on your ConvertKit dashboard, it will still be returned by the list API. I don't want to share these yet-to-be-sent newsletters on any of my sites, so we'll add some logic to getAllNewsletters to filter out any newsletters that haven't been published yet.
Don't render newsletters with duplicate subject lines
There are some cases where I send the same newsletter multiple times, with the same subject line, to different audiences - usually because I've forgotten to include some details in the original broadcast. This results in multiple broadcasts with essentially the same content. I don't want to show these on my site, so I added some logic to deduple any newsletters that have the same subject line, displaying only the most recent one (which should be the most correct, in theory).
This is the updated code for getAllNewsletters in convertKit.server.js:
1export const getAllNewsletters = async () => {2const response = await fetch(3`https://api.convertkit.com/v3/broadcasts?api_secret=${CONVERTKIT_API_SECRET}`4);5const data = await response.json();6const { broadcasts } = data;78// get details for each individual newsletter9const newsletters = await Promise.all(10broadcasts.map((broadcast) => getNewsletter(broadcast.id))11);1213// hackish dedupe here - sometimes we publish a newsletter a _second_ time as a correction, or to a different audience.14// In those cases, they should always have the same subject. We're using subject as a way to deduplicate newsletters in that case15const dedupedBySubject = {};1617// return only newsletters that have been published, and sort by most recent to oldest18newsletters19.filter((newsletter) => !!newsletter.published_at)20.sort((a, b) => new Date(b.published_at) - new Date(a.published_at))21.forEach((newsletter) => {22// the first one we encounter in this order will be the newest, so if there's one already we don't make any changes to the object map23if (!dedupedBySubject[newsletter.subject])24dedupedBySubject[newsletter.subject] = newsletter;25});2627let nls = [];28// iterate over the map we have of subject->newsletter, and push into a fresh array, which we'll return29for (let subject in dedupedBySubject) {30nls.push(dedupedBySubject[subject]);31}3233return nls;34};
And with that, our journey is complete - we've created a /newsletter page that shows a list of all of our newsletters, with a thumbnail image for each one. We've also added a /newsletter/$id page that shows the full content of a given newsletter.
Wrap-up
I hope this post has been helpful to you, and that you've learned something new about how to use ConvertKit's API to create a custom newsletter index page for your Remix site. To see what this looks like in practice, head over to primaryfocus.tv/newsletter.
In my next post, I'll share how I used a similar implementation in Next.js on my site - mikebifulco.com.
If you have any questions, or if you've found a bug in my code, please feel free to reach out to me on Twitter @irreverentmike!