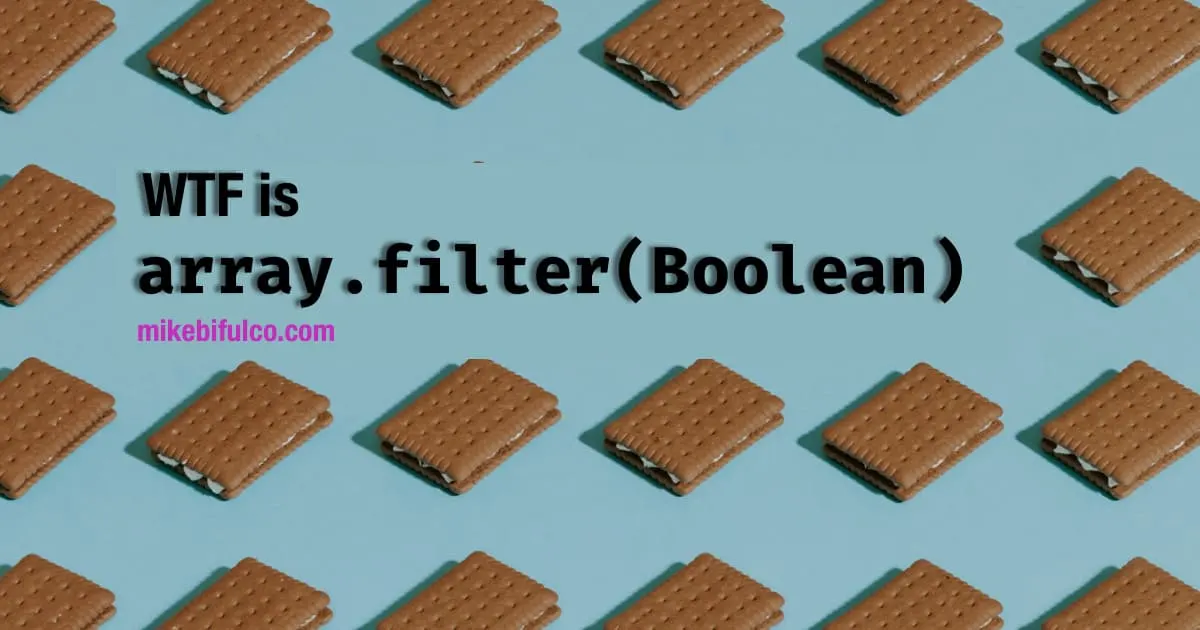
What does .filter(Boolean) do on Arrays?
This is a pattern I've been coming across quite a bit lately in JavaScript code, and can be extremely helpful once you understand what's going on. In short, it's a bit of functional programming which is used to remove null and undefined values from an array.
1const values = [1, 2, 3, 4, null, 5, 6, 7, undefined];23console.log(values.length);4// Output: 956console.log(values.filter(Boolean).length);7// Output: 789// note that this does not mutate the value original array10console.log(values.length);11// Output: 9
How does the Boolean part of .filter(Boolean) work?
We're using a function built into arrays in JavaScript, called Array.prototype.filter, which creates a new array containing all elements that pass the check within the function it takes as an argument. In this case, we're using the JavaScript Boolean object wrapper's constructor as that testing function.
Boolean is a helper class in JavaScript which can be used to test whether a given value or expression evaluates to true or false. There's a subtle, but really important point here - Boolean() follows the JavaScript rules of truthiness. That means that the output Boolean() might not always be what you imagine.
In this context, passing Boolean to .filter is effectively shorthand for doing this:
1array.filter((item) => {2return Boolean(item);3});
which is also approximately the same as
1array.filter((item) => {2return !!item; // evaluate whether item is truthy3});
or, simplified
1array.filter((item) => !!item);
I suspect that you may have seen at least one of these variations before. In the end, array.filter(Boolean) is just shorthand for any of the other options above. It's the kind of thing that can cause even seasoned programmers to recoil in horror the first time they see it. Near as I can tell, though, it's a perfectly fine replacement.
Examples of Boolean evaluating for truthiness
1// straightforward boolean2Boolean(true); // true3Boolean(false); // false45// null/undefined6Boolean(null); // false7Boolean(undefined); // false89// hmm...10Boolean(NaN); // false11Boolean(0); // false12Boolean(-0); // false13Boolean(-1); // true1415// empty strings vs blank strings16Boolean(''); // false17Boolean(' '); // true1819// empty objects20Boolean([]); // true21Boolean({}); // true2223// Date is just an object24Boolean(new Date()); // true2526// oh god27Boolean('false'); // true28Boolean('Or any string, really'); // true29Boolean('The blog of Mike Bifulco'); // true
Warning: Be careful with the truth(y)
So - someArray.filter(Boolean) is really helpful for removing null and undefined values, but it's important to bear in mind that there are quite a few confusing cases above... this trick will remove items with a value of 0 from your array! That can be a significant difference for interfaces where displaying a 0 is perfectly fine.
**EDIT: ** Hi, Mike from The Future™️ here - I've edited the next paragraph to reflect the actual truth... I had confused -1 with false from my days as a BASIC programmer, where we'd sometimes create infinite loops with while (-1)... but even that means "while true"!
I also want to call some attention to cases that evaluate to -1. for many programmers, -1 is synonymous with false, because that is absolutely the case in many langauges... but not here. The -1 case can also be unintuitive if you're not expecting it, but true to form, in JavaScript, -1 is a truthy value!
Array.filter(Boolean) For React Developers
I tend to come across this pattern being used fairly often for iterating over collections in React, to clean up an input array which may have had results removed from it upstream for some reason. This protects you from scary errors like Can't read property foo of undefined or Can't read property bar of null:
12const people = [3{4name: 'Mike Bifulco',5email: 'hello@mikebifulco.com',6},7null,8null,9null,10{11name: "Jimi Hendrix",12email: 'jimi@heyjimihimi@guitarsolo',13}14]1516// display a list of people17const PeopleList = ({people}) => {18return (19<ul>20{people.map(person) => {21// this will crash if there's a null/undefined in the list!22return (23<li>{person.name}: {person.email}</li>24);25}}26</ul>27);28}2930// a safer implementation31const SaferPeopleList = ({people}) => {32return (33<ul>34{people35.filter(Boolean) // this _one weird trick!_36.map(person) => {37return (38<li>{person.name}: {person.email}</li>39);40}41}42</ul>43);44}
Functional Programming reminder
Like I mentioned above, this is a handy bit of functional programming -- as is the case with nearly all clever bits of functional programming, it's important to remember that we're not mutating any arrays here - we are creating new ones. Let's show what that means in a quick example:
1const myPets = [2'Leo',3'Hamilton',4null,5'Jet',6'Pepper',7'Otis',8undefined,9'Iona',10];1112console.log(myPets.length); // 81314myPets15.filter(Boolean) // filter null and undefined16.forEach((pet) => {17console.log(pet); // prints all pet names once, no null or undefined present18});1920console.log(myPets.length); // still 8! filter _does not mutate the original array_
Wrapping up
Hopefully this has helped to demystify this little code pattern a bit. What do you think? Is this something you'll use in your projects? Are there dangers/tricks/cases I didn't consider here?
Tell me all about it on twitter @irreverentmike. Thanks for reading!
note: Cover photo for this article is from Pawel Czerwinski on Unsplash